Styling Options
Customization basics
The following topic covers a bunch of customization options you can implement in either the Frontegg Embedded or Hosted modes.
The loginBox
attribute allows you to customize style elements at your login-box level and provides additional flexibility for the sub-attributes and pages linked to it (such as activate account
, forgot password
, MFA
etc.).
Changing the logo
Changing the default logo is a great example of customizing your login box appearance. To change your logo, insert your desired image within the logo
property of your themeOptions
object, like so:
themeOptions: FronteggThemeOptions = {
loginBox: {
logo: {
image: 'https://assets.frontegg.com/public-frontegg-assets/acme-logo.svg'
},
}
}
Which results in the following:
Changing Default Styling Examples
By default, the Frontegg login box has a modern
theme that looks like this:
To change the theme name programmatically, you can do so under the themeName
key:
const themeOptions: FronteggThemeOptions = {
loginBox: {
themeName: 'classic',
}
}
In the above case, the classic
theme overrides the default and results in the following:
Available Themes
The theme names you can use are: modern, classic, vivid, and dark.
Changing the browser title
Changing the browser title is available under the docTitle
key, like so:
//Required versions:
//@frontegg/[email protected]
//@frontegg/[email protected]
//@frontegg/[email protected]
//@frontegg/[email protected]
const themeOptions: FronteggThemeOptions = {
loginBox: {
login:{
docTitle: 'Login browser title'
},
signup: {
docTitle: 'Signup browser title'
}
}
}
Resulting in the following:
Migrating from older versions?
If you are migrating from older versions, this replaces the
headerImage
property used on theFronteggAppOptions
.
Changing the background image
Changing the background image of the entire page is available by using the rootStyle
attribute of the loginBox
:
import backgroundImage from '../assets/gradient';
const themeOptions: FronteggThemeOptions = {
loginBox: {
rootStyle: {
backgroundImage: backgroundImage
}
}
}
Which looks like:
Migrating from older versions?
If you are migrating from older versions, this replaces the
backgroundImage
property used on theFronteggAppOptions
.
Flat design
To control the appearance of the box, you can change the boxStyle
attribute within your loginBox
:
const themeOptions: FronteggThemeOptions = {
loginBox: {
boxStyle: {
boxShadow: 'none',
background: 'transparent'
},
}
}
Which looks like:
Adding a footer to the login box
In some use cases, you'd want to add a footer that includes your terms of use and privacy policy.
Adding such a footer at the login-box level will cause the footer to appear in all the login-box pages. Here is an example:
const themeOptions = {
loginBox: {
boxFooter: () => {
return (
<div
style={{
textAlign: 'center',
marginTop: '30px',
fontSize: '12px',
lineHeight: '16px',
color: '#36373C',
}}>
By continuing up I agree to Acme's{' '}
<a
target='_blank'
rel='noopener noreferrer'
style={{ color: '#36373C' }}
href={'https://acme.com/terms'}>
Terms of Service
</a>{' '}
and{' '}
<a
target='_blank'
rel='noopener noreferrer'
style={{ color: '#36373C' }}
href={'https://acme.com/policy'}>
Privacy Policy.
</a>
</div>
)
}
}
}
Which generates the following:
⚡️ The same footer can be applied only to a specific page, e.g., the Signup page, by moving it under the loginBox
section as done below:
const themeOptions: FronteggThemeOptions = {
loginBox: {
signup: {
boxFooter: () => {
return (
<div>
...
</div>
)
}
}
}
}
Which removes it from the Sign in
page and adds it to the Sign up
page:
Additionally, you can place the footer outside of the login box (on the page) as done below:
const themeOptions: FronteggThemeOptions = {
loginBox: {
signup: {
pageFooter: () => {
return (
<div>
...
</div>
)
}
}
}
}
Which generates the following:
Split layout for the login box
In some of the cases, you will choose to have the login and signup forms not in the center of the dialog, but split with some marketing or commercials messaging on the right of it.
The way to achieve it is via the layout
property of the loginBox
as the snippet below shows.
const themeOptions: FronteggThemeOptions = {
loginBox: {
layout: {
type: 'float-left',
splitSize: 50,
sideElement: () => {
return <div style={{width: '50%'}}>
<img src="https://images.unsplash.com/photo-1526374965328-7f61d4dc18c5?ixid=MnwxMjA3fDB8MHxwaG90by1wYWdlfHx8fGVufDB8fHx8&ixlib=rb-1.2.1&auto=format&fit=crop&w=2370&q=80"
alt="Hackers"/>
</div>
},
sideElementStyle: {
width: '50%'
}
}
}
}
Which results in the following:
Customizing the social login buttons
By default, the social login buttons are spread horizontally on the same row.
You can change the way that they are spread from a row
flex mode to a column
flex as done below:
const themeOptions: FronteggThemeOptions = {
loginBox: {
socialLogins: {
containerStyle: {
display: 'flex',
flexDirection: 'column',
},
buttonStyle: {
base: {
marginTop: '10px'
}
}
},
}
}
Which orders the buttons like this:
Removing the text from the buttons
If you want to remove the text of the social login buttons and leave it with icons only, this is possible as well using the snippet below:
const themeOptions: FronteggThemeOptions = {
loginBox: {
socialLogins: {
iconsOnly: true,
},
}
}
Which looks like this:
Changing the colors of the social login buttons
You can control the colors of the social login buttons by overriding the buttonStyle
property on the socialLogins
buttons section:
const themeOptions: FronteggThemeOptions = {
loginBox: {
socialLogins: {
buttonStyle: {
base: {
borderRadius: '0.5rem',
color: '#000',
background: '#fff',
border: '0.5px solid black'
}
},
},
}
}
Which looks like this:
Controlling the social logins divider
You can change the style of the divider using the dividerStyle
attribute under the socialLogins
property of the loginBox
:
const themeOptions: FronteggThemeOptions = {
loginBox: {
socialLogins: {
dividerStyle: {
backgroundColor: '#f5f5f5',
borderColor: '#f5f5f5',
borderWidth: '1px',
borderStyle: 'solid',
marginTop: '10px',
marginBottom: '10px',
}
},
}
}
Which results the following:
Not enough? Want to implement your own divider? We got you covered! 🚀
import React from "react";
import contextOptions from './context-options';
import {FronteggProvider, FronteggThemeOptions} from "@frontegg/react";
const themeOptions: FronteggThemeOptions = {
loginBox: {
socialLogins: {
divider: () => {
return <div>My super duper divider</div>
},
},
}
}
const Provider = () => (
<FronteggProvider contextOptions={contextOptions} themeOptions={themeOptions}>
<div />
</FronteggProvider>
);
export default Provider;
Customizing the inputs
Customizing the style of the inputs on the login box is available using the inputTheme
under the loginBox
section:
const themeOptions: FronteggThemeOptions = {
loginBox: {
inputTheme: {
base: {
backgroundColor: '#f5f5f5',
borderColor: '#e5e5e5',
color: '#333',
borderRadius: '4px',
borderWidth: '1px',
borderStyle: 'solid',
boxShadow: '0 0 0 1px rgba(0,0,0,.1)',
fontSize: '14px',
fontWeight: '400',
height: '40px',
padding: '0 15px',
transition: 'border-color .2s ease-in-out, box-shadow .2s ease-in-out',
},
}
}
}
Which looks like this:
Customizing the links
The links (such as the Don't have an account? Sign up
) on the login box can be customized as well:
const themeOptions: FronteggThemeOptions = {
loginBox: {
linkButtonTheme: {
base: {
color: 'red',
textDecoration: 'underline',
fontWeight: 'bold',
}
},
}
}
Which looks like this:
Customizing the submit button
The submit (login) button can be customized using the snippet below:
const themeOptions: FronteggThemeOptions = {
loginBox: {
submitButtonTheme: {
base: {
backgroundColor: '#00bcd4',
color: '#fff',
borderColor: 'transparent'
}
}
}
}
Which looks like this:
Changing the input errors
Style the inputs and labels for the error messages in your forms. For instance, change the color and font family. Here is sample code that changes the color
to pink and fontFamily
to monospace for the input error alerts:
const themeOptions: FronteggThemeOptions = {
components: {
MuiFormHelperText: {
styleOverrides: {
root: {
"&.Mui-error": {
color: "pink",
fontFamily: "monospace",
},
},
},
},
}
}
Which looks like this:
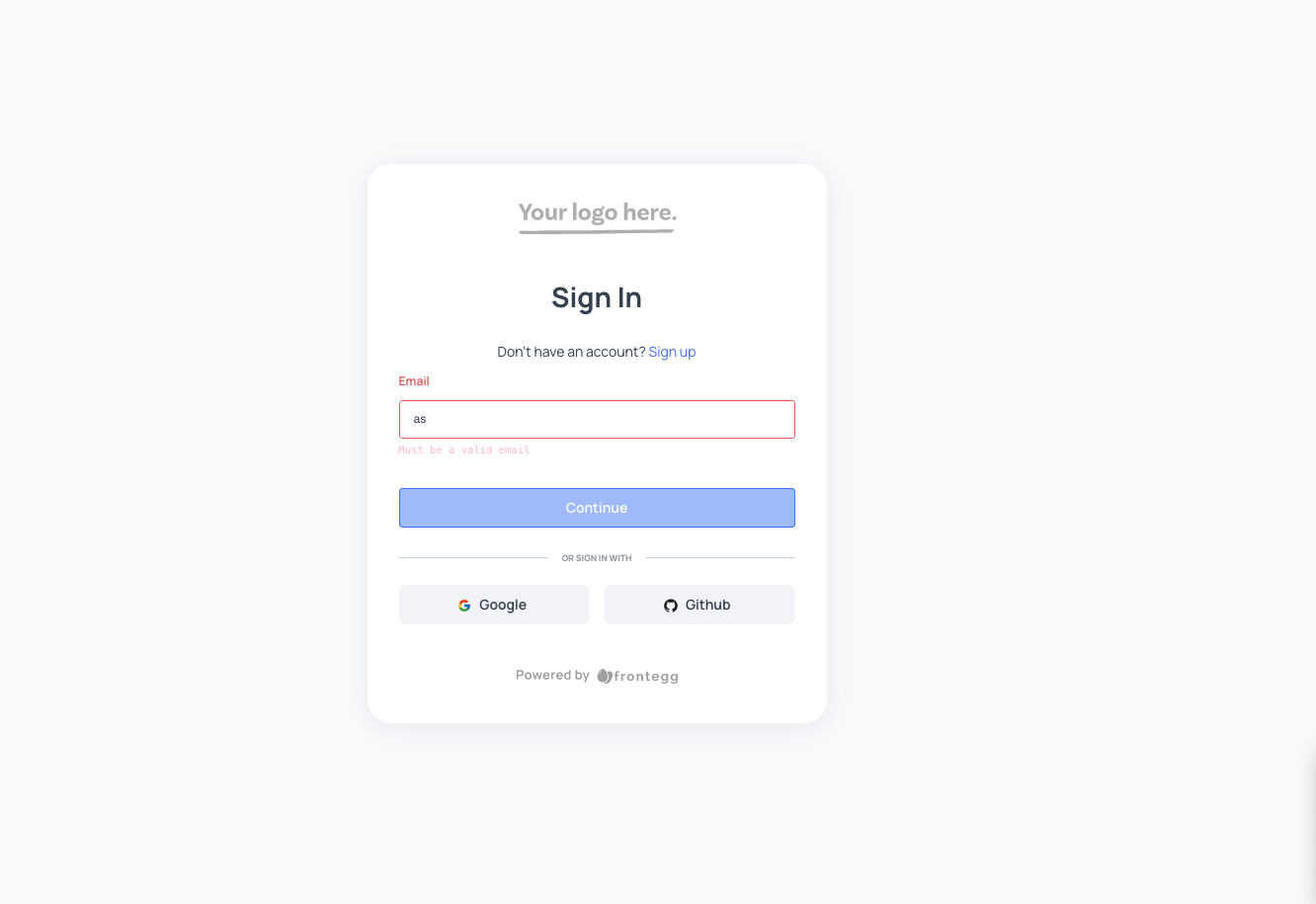
Customizing the font globally
Set the fontFamily
at the global level to monospace
:
const themeOptions = {
typographyStyleOptions: {
fontFamily: 'staatliches',
},
}
Customizing the loader
The loader we display when loading the login box can be customized as well. You actually can implement your own loader and get the loading
hook from the application provider in order to know when to display it. Just as shown in the snippet below. In this example, we utilized a loader element from semantic-ui):
import React, {useState} from "react";
import {FronteggProvider} from "@frontegg/react";
import {Dimmer, Loader} from "semantic-ui-react";
import contextOptions from './context-options';
// Replace this with your app logo 👇
const headerImage = 'https://assets.frontegg.com/public-frontegg-assets/acme-logo.svg';
const MyCustomLoaderComponent = () => {
return (
<Dimmer active>
<Loader>Loading using custom loader!</Loader>
</Dimmer>
)
}
const Provider = () => {
const [loading, setLoading] = useState(true);
return (
<div>
<FronteggProvider customLoader={setLoading} contextOptions={contextOptions} headerImage={headerImage}>
<div />
</FronteggProvider>
{loading && <MyCustomLoaderComponent />}
</div>
)
};
export default Provider;
Which looks like this:
Endless opportunities exist. Check out the examples below and visit our interactive code sandbox.
GitHub code samples
⚡️ Visit our Frontegg Samples repository in GitHub ⚡️
Updated 3 months ago