Frontend Integration
The next step of integration will be to embed the Frontegg SSO component in order to allow your customers to configure SSO via a self-service approach
React support
The embed integration of the SSO component is currently supported for React only
For that to happen we will need to install the Frontegg frontend library on your frontend project:
yarn add react-router-dom @frontegg/react-core @frontegg/react-auth
npm i react-router-dom @frontegg/react-core @frontegg/react-auth
Add a file called withFrontegg.js
to your application to be the High Order Component which will wrap your application (change the [YOUR-API-GW-URL]
with the URL of your backend application which you installed the frontegg SDK on).
import React from 'react';
import { FronteggProvider } from '@frontegg/react-core';
import { AuthPlugin } from '@frontegg/react-auth';
/**
* use this object to config Frontegg global context object
*/
const contextOptions = {
baseUrl: '[YOUR-API-GW-URL]',
requestCredentials: 'include',
};
const plugins = [
// add frontegg plugin here
AuthPlugin({
}),
];
export const withFrontegg = (AppComponent) => (props) => {
return <FronteggProvider
plugins={plugins}
context={contextOptions}>
<AppComponent {...props} />
</FronteggProvider>;
};
Wrap your application with the withFrontegg wrapper
:
import React from 'react';
import './index.css';
import App from './App';
import ReactDOM from 'react-dom';
import { withFrontegg } from "./withFrontegg";
const AppWithFrontegg = withFrontegg(App);
ReactDOM.render(
<div>
<AppWithFrontegg />
</div>,
document.getElementById('root')
);
And add the Frontegg SSO component
on the relevant page you want to host it:
import React from 'react';
import { SSO } from '@frontegg/react-auth';
function SamlPage() {
return (
<React.Fragment>
<SSO.Page />
</React.Fragment>
)
}
export default SamlPage;
The SSO component should now be available for your customers to configure.
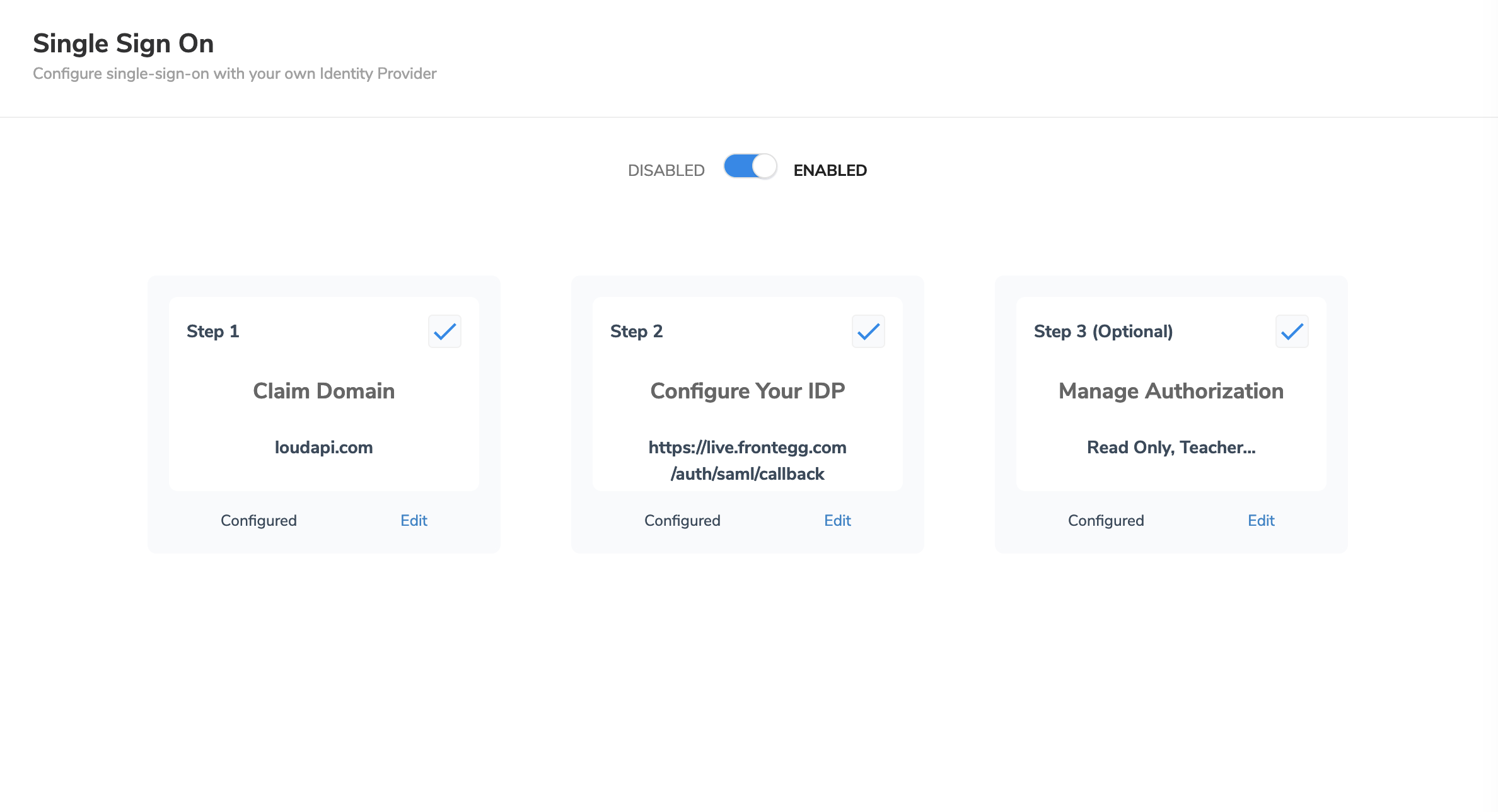
Non customer-facing configuation
Frontegg offers postman collections in case you don't want to expose the SSO configuration to your customers and would like to configure the SSO flows on your own.
Please contact us at [email protected] to get access to these collections.
Updated 5 months ago