Backend Integration
First Get Your API Key and Client ID
Once you signup with Frontegg, an API Key and Client ID are automatically generated for you. To retrieve them, simply login to the Frontegg Portal and open the Administration/General menu.
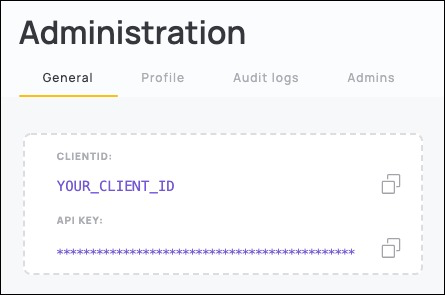
Node.JS
STEP 1: Create a New Node.js Project
If you have an existing app, skip this step.
Under the root of the project, create a folder named backend
(under the src
folder).
mkdir backend
cd backend
npm init -y
npm i express cors @frontegg/client
touch index.js
STEP 2: Update index.js
Use the following code to update the server file.
const express = require('express');
const app = express();
const cors = require('cors');
const { frontegg, withAuthentication, FronteggPermissions } = require('@frontegg/client');
const clientId = process.env.FRONTEGG_CLIENT_ID || 'YOUR-CLIENT-ID';
const apiKey = process.env.FRONTEGG_API_KEY || 'YOUR-API-KEY';
app.use(cors({ origin: 'http://localhost:3000', credentials: true }))
app.use('/frontegg', frontegg({
clientId,
apiKey,
authMiddleware: withAuthentication(),
contextResolver: req => {
return {
tenantId: req.user ? req.user.tenantId : '',
userId: req.user ? req.user.id : '',
permissions: [FronteggPermissions.All],
}
}
}));
app.listen(8080);
Frontegg is now integrated with your backend. You can run it now.
node index
python server.py
Python
STEP 1: Create a New Python Project
If you have an existing app, skip this step.
Under the root of the project, create a folder named backend
(under the src
folder).
mkdir backend
cd backend
pip install flask flask_cors frontegg
cd backend
touch server.py
mkdir backend
cd backend
pip install fastapi frontegg
cd backend
touch server.py
mkdir backend
cd backend
pip install sanic sanic_cors frontegg
cd backend
touch server.py
STEP 2: Update server.py
Use the following code to update the server file.
import os
from flask import Flask
from frontegg.flask import frontegg
from frontegg.flask.secure_access import context_provider
from frontegg import FronteggContext, FronteggPermissions
from flask_cors import CORS
app = Flask('test')
CORS(app, origins=["http://localhost:3000"], supports_credentials=True)
app.config['FRONTEGG_CLIENT_ID'] = os.environ.get('FRONTEGG_CLIENT_ID')
app.config['FRONTEGG_API_KEY'] = os.environ.get('FRONTEGG_API_KEY')
frontegg.init_app(app,
app.config['FRONTEGG_CLIENT_ID'],
app.config['FRONTEGG_API_KEY'], context_provider)
if __name__ == '__main__':
app.run(host='0.0.0.0', port=8080, debug=True)
from frontegg.fastapi import frontegg
from frontegg.fastapi.secure_access import FronteggSecurity, User, context_provider_with_permissions
from fastapi import FastAPI, Depends
from fastapi.middleware.cors import CORSMiddleware
import uvicorn
app = FastAPI()
client_id = 'my-client-id'
api_key = 'my-api-key'
frontegg.init_app(app, client_id=client_id, api_key=api_key, with_secure_access=True, context_provider=context_provider_with_permissions)
origins = [
"http://localhost:3000",
]
app.add_middleware(
CORSMiddleware,
allow_origins=origins,
allow_credentials=True,
allow_methods=["*"],
allow_headers=["*"],
)
uvicorn.run(app, port=8080)
from sanic import Sanic
import os
from frontegg.sanic import frontegg
from frontegg.sanic.secure_access import context_provider_with_permissions, with_authentication
from sanic_cors import CORS
from sanic.response import json
app = Sanic('test')
CORS(app, origins=["http://localhost:3000"], supports_credentials=True)
client_id = os.environ.get('FRONTEGG_CLIENT_ID')
api_key = os.environ.get('FRONTEGG_API_KEY')
frontegg.init_app(app, client_id, api_key, with_secure_access=True, context_provider=context_provider_with_permissions)
if __name__ == '__main__':
app.run(host='0.0.0.0', port=8080, debug=True)
Frontegg is now integrated with your backend. You can run it now.
python server.py
Updated 5 months ago